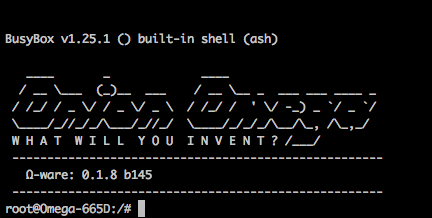
The second part of this week’s 2-Bullet Tuesday! See the first part here.
You can subscribe to the newsletter on the 2-Bullet Tuesday page!
Omega Tip of the Week:
Getting Started With git on the Omega
When developing code, it’s a great idea to use version control. Version control is a system of organizing and recording changes to files. git is one of the most popular version control systems, and it’s a great tool to learn when working with the Omega.
git can also be used to download code that others have made available over the Internet. We will cover how to do this on the Omega in the next issue. This time we’re covering how to use git to create a repo that is local to your Omega.
git Concepts
To keep track of changes to your code, you’ll be following this general process:
- Write new code or update existing code in your file(s).
- Add these changes to be staged (temporarily stored) by git.
- Repeat the above 2 steps until you’re ready to commit (lock in) all changes to the files’ change history, or remove changes if you’ve changed your mind.
- Commit the changes to your code and add them to your code’s change history.
Installing git
Make sure your Omega is on the latest firmware, then run these commands below:
opkg update opkg install git
Create a Repository
A git repository, or repo for short, is a single directory containing files and other directories that you want git to keep track of. On the Omega, you would typically keep separate projects in their own repos.
To initialize a new repo in an existing folder, run:
git init
To initialize a new repo in an existing directory, run:
git init (EXISTING DIRECTORY)
Let’s do this on the Omega. Run:
git init ~./myRepo cd ~/myRepo
Make Some Changes
We’ll first create a file called README.md and put a simple echo statement in it. Run:
echo "Example git repo" > README.md
Now check the status of repository by running git status. You should see:
On branch master Initial commit Untracked files: (use "git add (file..." to include in what will be committed) README.md nothing added to commit but untracked files present (use "git add" to track)
This means we need to add these changes to be staged. Run git add README.md to add the changes we just made. Then run git status again and you should see something like this:
On branch master Initial commit add Changes to be committed: (use "git rm --cached (file)..." to unstage) new file: README.md
Commit Your Changes
To commit these changes to the file history, run git commit -m “created README”. The -m flag is so you can add a brief message describing your changes to the files.
You should see something like the following:
[master (root-commit) d132183] created README Committer: root (snip) 1 file changed, 1 insertion(+) create mode 100644 README.md
We can ignore the warnings about username and hostname for now. Run git status again and you’ll see the following:
On branch master nothing to commit, working tree clean
This means that the changes recorded in our repository are up-to-date with the source files.
Repeat this process by creating a script file with a simple echo statement like below:
echo "echo 'Hello world!'" >> myScript.sh echo "echo 'I like cats'" >> myScript.sh git add myScript.sh git commit -m "I like cats"
If you run this script, you should see:
Hello world! I like cats
Branching
What if we wanted to modify our code, but still be able to revert back to a clean working state? We can use branches to help us here.
File changes are saved to branches which can be combined with other branches to produce the most up-to-date version of your code. The default branch is master, and is typically used for production, deployment-ready code. Development usually takes place in other branches such as dev to prevent incomplete or buggy code from affecting anything that has been released for use.
To switch between branches, use the git checkout command. To create a new branch, run git checkout -b [new branch].
Create a new branch called dogs:
git checkout -b dogs
Now run the following command to replace the ‘cats’ with ‘dogs’ in the script:
sed -i 's/cats/dogs/' myScript.sh
Your script should now print:
Hello world! I like dogs
Run the following to add a new commit to the dogs branch:
git add myScript.sh git commit -m “Actually I like dogs”
If we’re satisfied with these changes, we can merge them back into the master branch. (If you’re more of a cat person, you can skip this step to leave your master code intact)
git checkout master git merge dogs
If you merged the changes from the dogs branch into master, you probably want to delete it. Switch to any other branch (like master), then run git branch -d [branch] like so:
git branch -d dogs
If you haven’t merged it, you may want to keep it handy in case you want to revisit or fix it for later.
Branches are useful when adding new features to code that’s already running properly so that you can always revert back to a working state.
And that’s it! Next week, we’ll explain how to work with remote repositories so that you can upload your code on the Internet, which is another neat way to update code wirelessly on the Omega. Or, you can also download code made public by other developers around the world!
Let us know what kind of stuff you would like to see featured on 2–Bullet Tuesday! Send a tweet to @OnionIoT with your suggestions!
Thanks for reading! Have a great week!